Understanding How to Parse Query String in Web Development
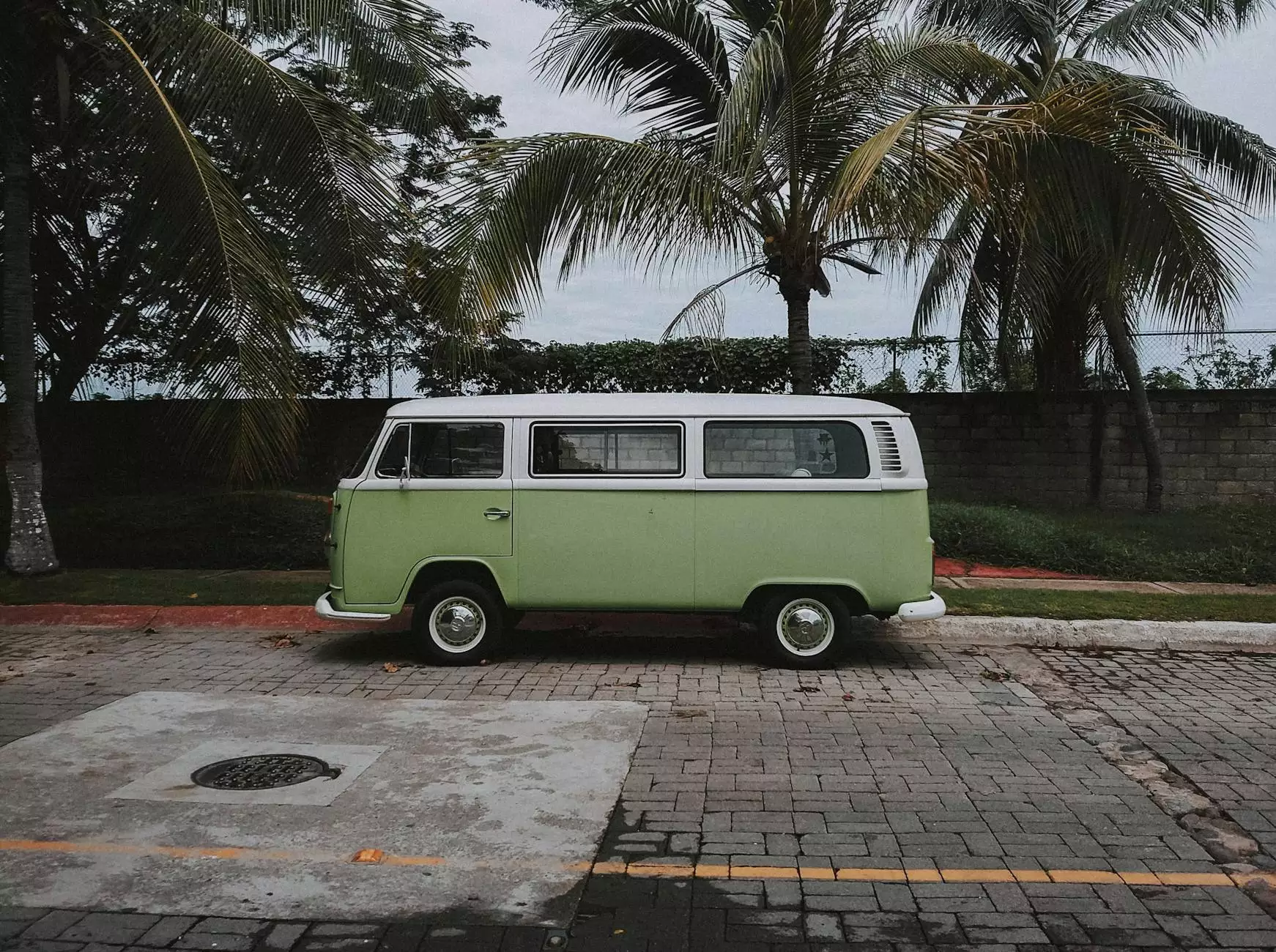
In the realm of web development and software development, efficiently managing data transmission is crucial for creating dynamic and interactive applications. One of the fundamental concepts in this area is how to parse query strings. This article will thoroughly explore what query strings are, the significance of parsing them, and the methodologies involved in parsing them effectively. Additionally, we will illustrate practical examples that can help web developers and business owners enhance their online applications.
What is a Query String?
A query string is a portion of a URL that contains data to be sent to a web server. It typically appears after the question mark (?) in a URL. For instance:
http://example.com/page?name=John&age=30In this example, the query string is name=John&age=30, consisting of two key-value pairs: name with value John, and age with value 30.
Why is Parsing Query Strings Important?
Parsing query strings is a vital process in web application development for several reasons:
- User Experience: By parsing query strings, developers can provide personalized experiences, such as tailor-making content based on user inputs.
- Data Handling: Websites often rely on query strings to transfer data between pages. Parsing these strings allows developers to handle user data efficiently.
- SEO Benefits: Correct management of query strings can enhance SEO performance by ensuring that search engines can correctly index pages.
- Security: Proper parsing can help mitigate vulnerabilities such as SQL injection by validating and sanitizing inputs.
How to Parse Query Strings?
Parsing a query string can be accomplished in several programming languages, but the basic methodology generally involves splitting the query string at ampersands (&) and then further splitting each key-value pair at the equals sign (=). Below, we present examples in various popular programming languages:
Parsing Query Strings using JavaScript
In JavaScript, you can use the URLSearchParams interface to easily parse query strings. Here’s a sample code snippet:
const url = new URL('http://example.com/page?name=John&age=30'); const params = new URLSearchParams(url.search); console.log(params.get('name')); // Outputs: John console.log(params.get('age')); // Outputs: 30Parsing Query Strings using Python
In Python, the urllib.parse module provides utilities for parsing URLs. Below is an example:
from urllib.parse import urlparse, parse_qs url = 'http://example.com/page?name=John&age=30' parsed_url = urlparse(url) query_params = parse_qs(parsed_url.query) print(query_params['name'][0]) # Outputs: John print(query_params['age'][0]) # Outputs: 30Parsing Query Strings using PHP
PHP has built-in support for parsing query strings through the $_GET superglobal. Here’s how it works:
parse_str($_SERVER['QUERY_STRING'], $query); echo $query['name']; // Outputs: John echo $query['age']; // Outputs: 30The Role of Query Strings in APIs
In the modern web, APIs play a crucial role in allowing different applications to communicate. Query strings are often used in APIs to provide parameters for requests. For instance, a weather API might use query strings to specify a location:
GET http://api.weather.com/v1/current?city=NewYork&unit=metric
Parsing the query string here helps the server understand what data to return. Properly managing these parameters ensures that developers can deliver accurate and efficient responses to API calls.
Best Practices for Managing Query Strings
To effectively use query strings within your web applications, consider the following best practices:
- Use Descriptive Parameter Names: Choose clear and descriptive names for your parameters to make it easy for users and developers to understand their purpose.
- Limit the Length of Query Strings: While browsers support long query strings, keeping them concise improves readability and performance.
- Consider Encoding: Use URL encoding to ensure that special characters do not interfere with the parsing process.
- Validate and Sanitize Inputs: Always validate and sanitize query string inputs to protect against attacks such as XSS or SQL injection.
- Utilize Frameworks and Libraries: Use existing frameworks or libraries to handle query string parsing to avoid reinventing the wheel and ensure best practices are followed.
Final Thoughts
In conclusion, understanding how to parse query strings effectively is essential for any web developer or business looking to create applications that thrive in an interactive online environment. By mastering this skill, developers can enhance user experience, streamline data handling, and contribute positively to overall site performance.
As you continue your web development journey, remember that the finer points of URL management, including query string parsing, can significantly impact how users interact with your application, ultimately reflecting on your business’s success. Whether you are designing a website or developing sophisticated software applications, always prioritize effective query string management in your development practices.
Explore More with Semalt.Tools
If you are looking to enhance your web design or software development capabilities, consider visiting Semalt.Tools. Our resources and tools can guide you in creating robust applications and achieving your business goals.